Here begins one of the most fantastic subjects in computer programming: repetition structures. We recommend that you have read the previous article about conditional structures.
Let’s get down to business!
What are repetition structures?
Also known as a loop, a repetition structure is a construction that programming languages provide so that the programmer can save work with repetitive code.
Imagine that we need to write an algorithm to print the message “Algol.dev” 10 times on the screen. The code below does just that:
1.println("Algol.dev");
2.println("Algol.dev");
3.println("Algol.dev");
4.println("Algol.dev");
5.println("Algol.dev");
6.println("Algol.dev");
7.println("Algol.dev");
8.println("Algol.dev");
9.println("Algol.dev");
10.println("Algol.dev");
The problem with this type of code is its scalability (growth capacity). Imagine that, instead of 10, we need to print the message 1000 times. The code would be impractical to write!
To solve this type of problem, repetition structures arose. See in the code below how the message could be written 1000 times using a generic repetition structure (illustrative only):
1.structure(repeat 1000){
2. println("Algol.dev");
3.}
The repetition structure is a scope that provides a control for the repetition of a command or set of commands. In line 2 (code above) the message “Algol.dev” is automatically repeated 1000 times, as it is within the scope of a repetition structure.
The while statement
In most programming languages, repetition structures are implemented through the while statement.
The code below illustrates the structure of the while statement:
1.while(conditional expression){
2. // commands to be repeated
3.}
It works as follows: the conditional expression (line 1 of the code above) is tested and, “while” returns TRUE, the commands (line 2 of the code above) are repeated. The moment the conditional expression returns a FALSE value, the commands are no longer repeated.
See the code below for an example of printing numbers from 1 to 10, using the while command:
1.int count=1;
2.while(count <= 10){
3. println(count);
4. count++;
5.}
Note that the “count” variable is a control variable that is used by the while statement to control the repetitions.
The command “count++” (line 4 of the code above) increases the value of the control variable with each new repetition. When the value of “count” reaches 11, the expression “count <= 10” (line 2 of the code above) returns FALSE and repetitions are interrupted.
Click on the animation below and see the execution simulation of the algorithm above.
See this complete code below in several different programming languages:
- Java
- C
- C++
- C#
- Python
1.import java.util.Scanner;
2.class Main {
3. public static void main(String[] args) {
4. int count=1;
5. while(count <= 10){
6. System.out.println(count);
7. count++;
8. }
9. }
10.}
1.#include <stdio.h>
2.int main(void) {
3. int count=1;
4. while(count <= 10){
5. printf("%d\n",count);
6. count++;
7. }
8.}
1.#include <iostream>
2.int main() {
3. int count=1;
4. while(count <= 10){
5. std::cout << count << std::endl;
6. count++;
7. }
8.}
1.using System;
2.class MainClass {
3. public static void Main (string[] args) {
4. int count=1;
5. while(count <= 10){
6. Console.WriteLine(count);
7. count++;
8. }
9. }
10.}
1.count=1
2.while count <= 10:
3. print(count)
4. count=count+1
Exercise 01
Show that you understood the basic mechanics of how a repetition structure works. Identify the values generated by the algorithms.
Intervals recognition of the while statement
The greatest skill that a programmer should have when it comes to repetitions is the ability to quickly identify their value intervals.
To exemplify, let’s go back to the code from the previous section:
1.int count=1;
2.while(count <= 10){
3. println(count);
4. count++;
5.}
It is easy to see that the value of the control variable “count” starts at 1. It is also easy to observe by the expression “count <= 10” that the repetitions end when the value of “count” exceeds the value 10. And by the expression “count++“, we observe that the variable “count” is incremented every 1 unit.
Therefore, we can conclude that this repetition structure imposes an interval of 10 repetitions (from 1 to 10 with 1 step of increment). The illustration below details the interval recognition of the above algorithm:

A second example of the while statement
Let’s take a different example:
1.int count=3;
2.while(count <= 11){
3. println(count);
4. count=count+2;
5.}
It is easy to see that the value of the control variable “count” starts at 3. It is also easy to observe by the expression “count <= 11” that the repetitions end when the value of “count” exceeds the value 11. And by the expression “count=count+2“, we observe that the variable “count” is increased every 2 units.
Therefore, we can conclude that this repetition structure imposes an interval of 5 repetitions (from 3 to 11 with 2 steps of increment). The illustration below details the interval recognition of the above algorithm:
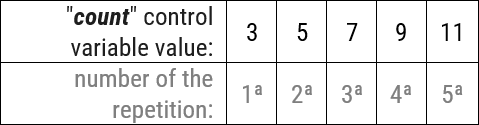
A more hard example
This example is more challenging. The control variable decreases instead of increasing:
1.int count=30;
2.while(count > 2){
3. println(count);
4. count=count-7;
5.}
We can see that the value of the control variable “count” starts at 30. It is also easy to observe by the expression “count > 2” that the repetitions end when the value of “count” reaches 2 or less. And by the expression “count=count-7“, we observe that the variable “count” is decreased every 7 units.
Therefore, we can conclude that this repetition structure imposes an interval of 4 repetitions (from 30 to 9 with 7 steps of decrement). The illustration below details the interval recognition of the above algorithm:
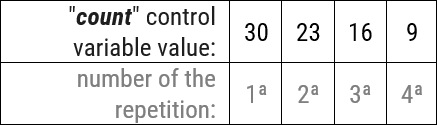
Exercise 02
Show that you understand the intervals recognition technique. Identify the intervals of repetition structures below.
Practices
Check out the practices we have prepared below to help you deepen your knowledge on this subject!
Choose your programming language and dig deeper.
Playlist
Continue your evolution on this subject and read our article about the “for” statement, the repetition structure most used by professional programmers.