Logical operators
Learn about the 4th category of programming operators. See how logical operators are used to create more complex conditional expressions.
11/07/2023
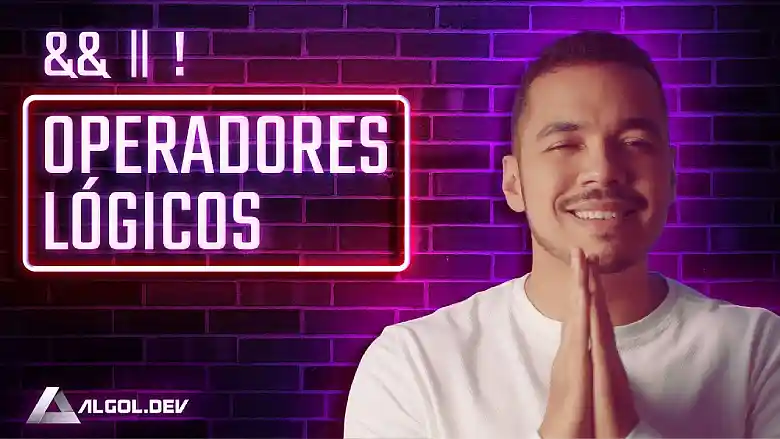
Before learning about logical operators, it is essential that you first know what relational operators are.
If you still don’t know what relational operators are, you should certainly read this article in which we explain in detail about the subject.
But let’s get down to business!
What are logical operators?
Logical operators represent the 4th category of programming operators and, in short, are used to create complex conditional expressions.
The main programming languages provide 3 logical operators:
&&
which represents the logical conjunction ‘and’.
||
which represents the logical disjunction ‘or’.
!
which represents the logical negation ‘not’.
However, it is important to note that in some programming languages these operators are given different symbols.
In Python, for example, operator symbols are used with their representation written in full in English:
and
for the logical conjunction.
or
for the logical disjunction.
not
for the logical negation.
These operators are used in order to create true or false expressions, but their operation is slightly more complex.
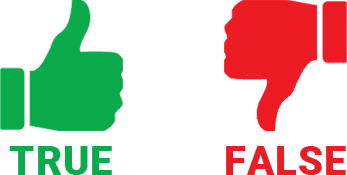
Use of the logical operator &&
First, you need to understand that these operators are generally used in conjunction with relational operators.
Just to illustrate, imagine that we want to create an algorithm to check if a person is of legal age. In this sense, we would have the code below:
1.if(age > 18){
2. println("You can access the system!");
3.}
Now imagine that, in addition to verifying that the person is older, we also want to verify that their level access is greater than the value 10. The conditional expression for this verification would be something like:
levelAccess > 10
We can put this in the algorithm using the logical conjunction operator &&, in such a way that this operator will join the expressions “age > 18” and “levelAccess > 10” into a single complex expression.
So, we would have the code below.
1.if(age > 18 && levelAccess > 10){
2. println("You can access the system!");
3.}
Note that the logical operator && joins the expressions “age > 18” and “levelAccess > 10“. In other words, the message on line 2 (of the code above) will be printed only if both conditions are true!
We can generalize the use of logical operators with the following model:
[left] relational oper. [right] LOGICAL OPERATOR [left] relational oper. [right];
Thus, it is possible to notice that a logical operator has the objective of joining two different expressions (using relational operators) and assembling a more complex conditional expression.
&& operator rules
Certainly, you already understood that the logical operator && joins two conditional expressions. However, it is important to show how it works in detail.
Example 1
Observe in the algorithm below that the variables age and levelAccess are initialized with the values 10 and 15, respectively:
1.int age = 10;
2.int levelAccess = 15;
3.if(age > 18 && levelAccess > 10){
4. println("You can access the System!");
5.}
As a result, note that, in line 3, the expression “age > 18” will return a false value and that the expression “levelAccess > 10” will return a true value.
3.if(age > 18 && levelAccess > 10){
Thus, because one of the two is false, the expression as a whole is assessed as false. As a result, the command on line 4 will not be executed.
1.int age = 10;
2.int levelAccess = 15;
3.if(age > 18 && levelAccess > 10){// the whole expression is false
4. println("You can access the System!");// not executed
5.}
Example 2
Now observe the same algorithm with different values for the variables age and levelAccess:
1.int age = 25;
2.int levelAccess = 9;
3.if(age > 18 && levelAccess > 10){
4. println("You can access the System!");
5.}
As a result, note that (in line 3) the expression “age > 18” will return a true value and that the expression “levelAccess > 10” will return a false value.
3.if(age > 18 && levelAccess > 10){
Like example 1, because one of the two is false, the expression as a whole is evaluated as false.
1.int age = 25;
2.int levelAccess = 9;
3.if(age > 18 && levelAccess > 10){// the whole expression is false
4. println("You can access the System!");// not executed
5.}
Truth table for the logical operator &&
The expression using the logical operator && is only evaluated as true if both the left and right expressions are true.
Below is the truth table with all possible combinations of True and False results for the use of the && operator.
left expression | operator | right expression | result |
---|---|---|---|
V | && | V | V |
V | && | F | F |
F | && | V | F |
F | && | F | F |
Use of the logical operator ||
Known as the logical disjunction ‘or’, the operator || works much like the && operator. The difference is only in the evaluation rule.
Note in the example below that the variables age and levelAccess are initialized with values 10 and 15 (lines 1 and 2) and that the logical operator we use is || (line 3).
1.int age = 10;
2.int levelAccess = 15;
3.if(age > 18 || levelAccess > 10){
4. println("You can access the System!");
5.}
As a result, note that, in line 3, the expression “age > 18” will return a false value, and that the expression “levelAccess > 10” will return a true value.
3.if(age > 18 || levelAccess > 10){
Thus, because one of the two is true, the expression as a whole is assessed as true.
1.int age = 10;
2.int levelAccess = 15;
3.if(age > 18 || levelAccess > 10){// the whole expression is true
4. println("You can access the System!");// is executed
5.}
For the logical disjunction operator || it is enough that one of the two expressions (left or right) is true.
In other words: either one or the other must be true for the whole expression to be true.
Truth table for the logical operator ||
Certainly, you already understood that the expression using the logical operator || is evaluated as true if only one of the two expressions left or right is true.
Below is the truth table with all possible combinations of True and False results for using the || operator.
left expression | operator | right expression | result |
---|---|---|---|
V | || | V | V |
V | || | F | V |
F | || | V | V |
F | || | F | F |
Use of the logical operator !
Known as logical negation ‘not’, the operator ! works as an inverter of the logical result.
Let’s explain.
You can certainly see in the code below that the logical expression “age > 18” (line 2) returns a false value. As a result, the command on line 3 will not be executed.
1.int age = 10;
2.if(age > 18){// expression is false
3. println("You can access the System!");// does not executed
4.}
We can use the logical negation operator ! to invert the result of the expression as follows:
!(age > 18)
We put the logical expression in parentheses and insert the operator ! before the expression.
Therefore, we can insert the operator ! in the algorithm as follows:
1.int age = 10;
2.if( !(age > 18) ){
3. println("You can access the System!");
4.}
In this way, the logical value of the expression “age > 18” is inverted by the operator ! and the conditional statement return the true value.
1.int age = 10;
2.if( !(age > 18) ){// expression becomes true
3. println("You can access the System!");// is executed
4.}
That simple!
You have certainly learned the basics of logical operators.
However, logical operators represent the 4th of the 5 categories of programming operators. So finish your learning about operators by reading our article on compound operators.
David Santiago
Master in Systems and Computing. Graduated in Information Systems. Professor of Programming Language, Algorithms, Data Structures and Development of Digital Games.