Before learning about composite operators, it is first recommended that you have read the articles on:
If you are unfamiliar with these operators, you should certainly read these articles before proceeding.
But let’s get down to business!
What are compound assignment operators?
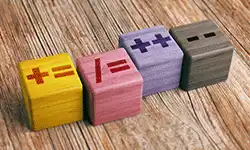
Compound assignment operators represent the 5th category of programming operators and, in summary, provide a shorter way to perform some arithmetic operations.
Just for example, see the code below. Note that, in line 2, the value assigned to variable x is 15. (10 + 5). Therefore, the command on line 3 prints the value 15 on the screen.
1.int x = 10;
2.x = x + 5;
3.println(x);
Now see below for another way to write that same code above.
1.int x = 10;
2.x += 5;
3.println(x);
You can certainly notice the use of a different operator in line 2 of the code above. We use the compound assignment operator += to simplify the arithmetic operation.
The expression “x = x + 5” contains two important characteristics:
- 1) Contains two simultaneous operations
- an assignment operation (x = x + 5)
- and an arithmetic operation (x = x + 5).
- 2) Use only a single variable in the same operation (the variable x).
Whenever an expression has these two characteristics, it can certainly be simplified with a compound assignment operator.
In this sense, the main programming languages provide the following compound assignment operators:
++
−−
which represents decrement.
+=
which represents attribution-addition.
−=
which represents attribution-subtraction.
*=
which represents attribution-multiplication.
/=
which represents attribution-division.
%=
which represents attribution-remainder.
Therefore, see below some examples of simplified expressions using the compound operators.
- The expression “x = x – 5” can be written as “x – = 5“
- In the same way “x = x * 8” can be written as “x * = 8“
- And “x = x / 2” can be written as “x / = 2“
Exercise 1
Show that you understand what compound assignment operators are and do the exercise below.
increment and decrement compound assignment operators
The ++ (increment) and −− (decrement) compound assignment operators are treated in a particular way. In fact, they are even simpler to understand.
The ++ (increment) operator only adds one unit to the value of the numeric variable.
For example: the expression “x = x + 1” could be rewritten as “x++“.
The −− (decrement) operator only subtracts one unit from the value of the numeric variable.
For example: the expression “x = x – 1” could be rewritten as “x−−“.
Very simple!
Exercise 2
Show that you understand the increment and decrement operators and do the exercise below.
Pre and post fixing of operators
The increment operator ++ and the decrement operator −− can be used in two different ways:
postfix – the operator is placed after the variable. Example: x++
prefix – the operator is placed before the variable. Example: ++x
Certainly, in most situations there will be no difference in using the postfix or prefix operator.
Just to illustrate, observe the codes below. In both examples, the value 4 for the variable x will be printed (in line 3).
1.int x = 3;
2.x++;
3.println(x);
1.int x = 3;
2.++x;
3.println(x);
Therefore, it does not make any difference to use the post or prefix increment operator in line 2 of the code above.
So, when does it make a difference?
The use of post and prefixing makes a difference when using ++ or −− in conjunction with an assignment operation. See an example below.
Note that there is an assignment operation (y = x ++) and an increment operation (y = x++).
Thus, the expressions “y = x++” and “y = ++x” generate different results.
Just to exemplify, observe the codes below. You can certainly see the difference between them on line 2.
1.int x = 3;
2.int y = x++;
3.println(y);
1.int x = 3;
2.int y = ++x;
3.println(y);
Surprisingly, in line 3 of the code above, different results are printed for the variable y.
The first prints the value 3 and the second prints the value 4.
But why does this happen?
It is all a matter of order of operations!
In the postfix expression “int y = x ++“, the value of x (value 3) is assigned to the variable y before the increment. That is:
- first the assignment is made (int y = x)
- then the increment is done (x++)
In the prefix expression “int y = ++x“, the value of x (value 3) is assigned to the variable y after the increment. That is:
- first the increment is done (++x)
- then the assignment is made (int y = x)
Very simple!
Exercise 3
Show that you understand the rules of postfix and prefix operators and do the exercise below.
Practices
Check out the practices we have prepared below to help you deepen your knowledge on this subject!
Choose your programming language and dig deeper.
Playlist
We hope this article has helped you to expand your knowledge.
If, in addition to this one, you also read all the previous articles on basic programming, you already have enough knowledge to move on to more complex subjects.
Try to delve into the universe of computer programming. Click here and access the second part of this Basic Programming course.