Conditional structures
What happens if you put one if-else structure inside the other? See the concept of conditional structures in this article.
11/09/2023
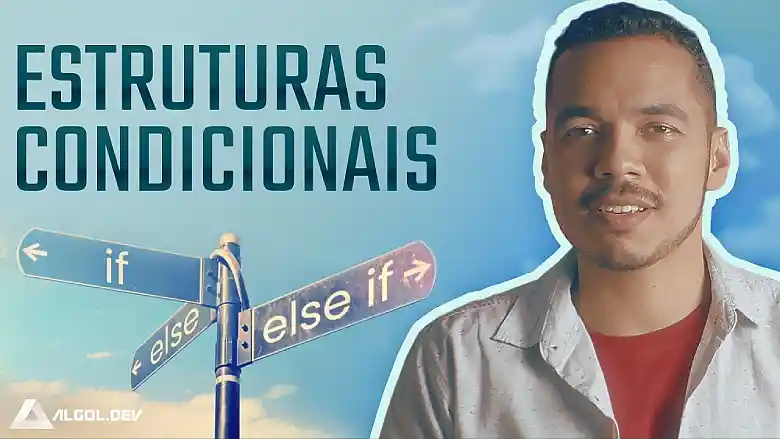
Here begins the second level of your learning in computer programming. We recommend that, before learning about conditional structures, you have already read all the articles in the first part of the basic programming course.
This article deals specifically with the evolution of the concept of “conditional statements”. If you still don’t know what conditional statements are, you should certainly read this article in which we explain in detail about the subject.
But let’s get down to business!
What are conditional structures?
When we want to program decision making in our algorithm, we use conditional statements; the if-else commands. Below is an example of a conditional in an algorithm that checks whether a student belongs to the CHILD category (from 0 to 11 years old):
1.if(age < 12){
2. println("Category: CHILD");
3.}
Now imagine that we need to add another category to our algorithm. If the student is not a CHILD, we still need to check if he belongs to the JUNIOR category (from 12 to 15 years old):
1.if(age < 12){
2. println("Category: CHILD");
3.} else {
4. if(age < 16){
5. println("Category: JUNIOR");
6. }
7.}
Notice in lines 4, 5 and 6 (code above) that we place a new conditional statement inside the first one. If the expression age < 12 (line 1 of the code above) returns false, the expression age < 16 (line 4 of the code above) will be evaluated.
Now imagine that we need to add another category. If the student is neither CHILD nor JUNIOR, we need to check if he belongs to the YOUNG category (from 16 to 19 years old):
1.if(age < 12){
2. println("Category: CHILD");
3.} else {
4. if(age < 16){
5. println("Category: JUNIOR");
6. } else {
7. if(age < 20){
8. println("Category: YOUNG");
9. }
10. }
11.}
Notice in lines 7, 8 and 9 (code above) that we place a new conditional statement, creating a series of nested if-else (one inside the other).
This is a CONDITIONAL STRUCTURE! A structure formed by conditional statements, one inside the other.
See below the final code of the algorithm, with the ADULT category already added:
1.if(age < 12){
2. println("Category: CHILD");
3.} else {
4. if(age < 16){
5. println("Category: JUNIOR");
6. } else {
7. if(age < 20){
8. println("Category: YOUNG");
9. } else {
10. println("Category: ADULT");
11. }
12. }
13.}
Optimization of conditional structures
Conditional structures suffer from a serious “readability” problem. Observe in the age category algorithm how the insertions of if-else within other if-else causes the code to grow to the right. Tending to a lying pyramid. Observe this phenomenon in the code below:
1.if(age < 12){
2. println("Category: CHILD");
3.} else {
4. if(age < 16){
5. println("Category: JUNIOR");
6. } else {
7. if(age < 20){
8. println("Category: YOUNG");
9. } else {
10. println("Category: ADULT");
11. }
12. }
13.}
This can become a huge problem if new conditions need to be added to the algorithm! Imagine that, instead of 4, we had ten categories?
To solve this problem, most programming languages implement OPTIMIZATION OF CONDITIONAL STRUCTURES. This optimization is applied through the “else if” command.
It works as follows: instead of creating the nested structure in the standard way:
1.if(expression 1){
2. // commands
3.} else {
4. if(expression 2){
5. // commands
6. }
7.}
We replaced the else command (line 3 of the code above) and the if command (line 4 of the code above) with the command “else if”. See below how the code looks using this optimization:
1.if(expression 1){
2. // commands
3.} else if(expression 2){
4. // commands
5.}
Note that this optimized structure has lost an indentation level, no longer showing the tendency of a lying pyramid.
It is also important to note that there may be some variations on this optimization in some programming languages. In the Python language, for example, the word “elif” is used in place of “else if”. See the example below:
1.if expression 1:
2. # commands
3.elif expression 2:
4. # commands
See below the complete code (in several programming languages) of the age category algorithm already optimized. Notice how it is leaner and more elegant.
1.import java.util.Scanner;
2.class Main {
3. public static void main(String[] args) {
4. System.out.println("Enter your age:");
5. Scanner scanner = new Scanner(System.in);
6. int age = scanner.nextInt();
7. if(age < 12){
8. System.out.println("Category: CHILD");
9. } else if(age < 16){
10. System.out.println("Category: JUNIOR");
11. } else if(age < 20){
12. System.out.println("Category: YOUNG");
13. } else {
14. System.out.println("Category: ADULT");
15. }
16. }
17.}
1.#include <stdio.h>
2.int main(void) {
3. printf("Enter your age:");
4. int age;
5. scanf("%d",&age);
6. if(age < 12){
7. printf("Category: CHILD");
8. } else if(age < 16){
9. printf("Category: JUNIOR");
10. } else if(age < 20){
11. printf("Category: YOUNG");
12. } else {
13. printf("Category: ADULT");
14. }
15. return 0;
16.}
1.#include <iostream>
2.int main() {
3. std::cout << "Enter your age:\n";
4. int age;
5. std::cin >> age;
6. if(age < 12){
7. std::cout << "Category: CHILD\n";
8. } else if(age < 16){
9. std::cout << "Category: JUNIOR\n";
10. } else if(age < 20){
11. std::cout << "Category: YOUNG\n";
12. } else {
13. std::cout << "Category: ADULT\n";
14. }
15.}
1.using System;
2.class MainClass {
3. public static void Main (string[] args) {
4. Console.WriteLine("Enter your age:");
5. int age = Convert.ToInt32(Console.ReadLine());
6. if(age < 12){
7. Console.WriteLine("Category: CHILD");
8. } else if(age < 16){
9. Console.WriteLine("Category: JUNIOR");
10. } else if(age < 20){
11. Console.WriteLine("Category: YOUNG");
12. } else {
13. Console.WriteLine("Category: ADULT");
14. }
15. }
16.}
1.age = int(input("Enter your age:"));
2.if age < 12:
3. print("Category: CHILD");
4.elif age < 16:
5. print("Category: JUNIOR");
6.elif age < 20:
7. print("Category: YOUNG");
8.else:
9. print("Category: ADULT");
Continue your evolution in programming and read our article on the while command, your beginning in the fantastic universe of repetition structures.
David Santiago
Master in Systems and Computing. Graduated in Information Systems. Professor of Programming Language, Algorithms, Data Structures and Development of Digital Games.