Function: modularization
Learn the technique of modularizing algorithms. A function make it possible to divide the source code into separate modules.
11/09/2023
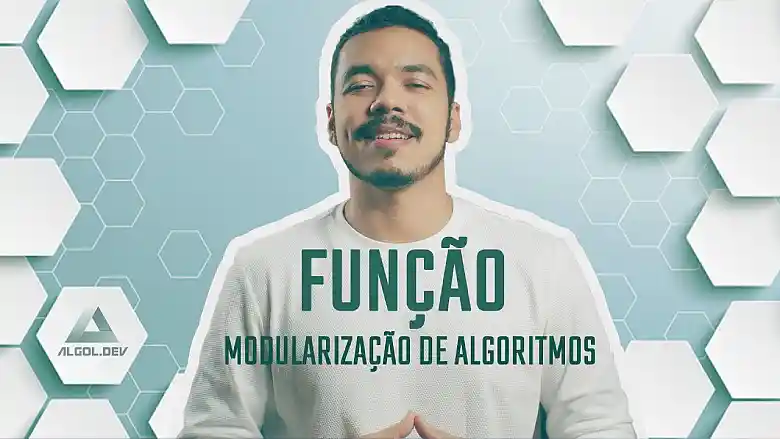
The concept of function is the last subject of basic programming that you must learn. It is recommended that, before reading this article, you already have the knowledge of the previous subjects. Therefore, it is worthwhile to click here and take a look at these other subjects. Maybe there are some that you missed?
But let’s get down to business!
Starting to understand a Function
The main purpose of modularization is to split the source code into smaller pieces, that do specific work and that are more manageable and easier to modify.
In computer programming, modularization is achieved by implementing a feature known as a function.
You can think of a function as a sequence of commands with a proper name. A kind of grouping of commands.
Let’s understand that!
Imagine that we have an algorithm that:
- Displays some welcome messages;
- Creates 3 variables;
- initializes the 3 variables with values entered by the user;
- Creates a 4th variable with the result of sum the 1st variable to the 2nd;
- Creates a 5th variable with the result of multiplying the 2nd variable with the 3rd;
- Prints the value of the 5 variables on the screen.
Observe the code of this algorithm below:
1.void main(){
2. // welcome
3. println("Hello");
4. println("Welcome to the System!");
5.
6. // creation of the 3 variables
7. int var1, var2, var3;
8.
9. // initialization
10. println("Enter a value:");
11. var1 = input();
12. println("Enter a value:");
13. var2 = input();
14. println("Enter a value:");
15. var3 = input();
16.
17. // sum operation
18. int var4 = var1 + var2;
19.
20. // multiplication operation
21. int var5 = var2 * var3;
22.
23. // variables printing
24. println("Value1: "+var1);
25. println("Value2: "+var2);
26. println("Value3: "+var3);
27. println("Value4: "+var4);
28. println("Value5: "+var5);
29.}
NOTE: The term “void main()” (line 1 of the code above) refers to the main function of the algorithm. In most programming languages, commands must be written within a main function, which represents the starting point of a program. However, it is important to note that there are exceptions, such as the Python and Javascript languages, which have no main function.
It is possible to notice that the previous algorithm performs 4 different types of activities:
- Request for values;
- An Sum operation;
- A Multiplication operation;
- And some prints.
It is obvious that the code above is simple to understand. But, depending on the size of the code, wouldn’t it be bad to leave many activities within the same scope? Wouldn’t it be interesting if there was a possibility to separate (modularize) these activities into separate blocks of code? Who knows even in other files?
And that is exactly what a function does! It allows you to separate parts of your algorithm into another code fragment, which can be in the same file or even in another file.
Let’s see how we can do this!
Creating a Simple Function
Let’s go back to the previous algorithm:
1.void main(){
2. // welcome
3. println("Hello");
4. println("Welcome to the System!");
5.
6. // creation of the 3 variables
7. int var1, var2, var3;
8.
9. // initialization
10. println("Enter a value:");
11. var1 = input();
12. println("Enter a value:");
13. var2 = input();
14. println("Enter a value:");
15. var3 = input();
16.
17. // sum operation
18. int var4 = var1 + var2;
19.
20. // multiplication operation
21. int var5 = var2 * var3;
22.
23. // variables printing
24. println("Value1: "+var1);
25. println("Value2: "+var2);
26. println("Value3: "+var3);
27. println("Value4: "+var4);
28. println("Value5: "+var5);
29.}
Imagine that we need to separate the printing of the welcome messages in another block of code (lines 3 and 4 of the code above).
For this, we can create a function called “welcome”. This function would only have the purpose of printing the messages. The code below shows this function:
1.void welcome(){
2. println("Hello");
3. println("Welcome to the System!");
4.}
Its structure may seem a little confusing at first, but let’s explain its components in detail:
- void – reserved word that indicates that the function does not return values (the concept of return will be detailed later).
- welcome – is the name of the function. It can be the name you prefer, as long as it represents the purpose of the function.
- ( ) – parentheses are the striking detail of a function. The function parameters are written inside them. If the function does not receive parameters, they are empty, but they must always exist (the parameters will be detailed later).
- { } – the Braces delimit the scope of the function. Within them we place all the commands that we want to separate from the main block of the algorithm.
This separation of code is what we call modularization!
Thus, the main code of the algorithm is as follows:
1.void welcome(){
2. println("Hello");
3. println("Welcome to the System!");
4.}
5.
6.void main(){
7. welcome();
8.
9. // creation of the 3 variables
10. int var1, var2, var3;
11.
12. // initialization
13. println("Enter a value:");
14. var1 = input();
15. println("Enter a value:");
16. var2 = input();
17. println("Enter a value:");
18. var3 = input();
19.
20. // sum operation
21. int var4 = var1 + var2;
22.
23. // multiplication operation
24. int var5 = var2 * var3;
25.
26. // variables printing
27. println("Value1: "+var1);
28. println("Value2: "+var2);
29. println("Value3: "+var3);
30. println("Value4: "+var4);
31. println("Value5: "+var5);
32.}
Notice in line 7 of the code above that the printing commands for the welcome messages have been removed. Now they are inside the body of the “welcome()” function (lines 1 to 4 of the code above).
The command welcome() (line 7 of the code above) represents what we call function invocation. He is responsible for diverting the execution of the algorithm to line 1 (code above) and printing the welcome messages.
Therefore, the creation of a function is divided into two parts:
- The function declaration – your body:
1.void welcome(){
2. println("Hello");
3. println("Welcome to the System!");
4.}
- The function invocation – the command that will divert the execution of the algorithm:
1.welcome();
See the animation below for a simulation of the execution, line by line, of the algorithm with the “welcome()” function.
Creating a function with a Return
Let’s go back to the same previous algorithm, from where we left off:
1.void welcome(){
2. println("Hello");
3. println("Welcome to the System!");
4.}
5.
6.void main(){
7. welcome();
8.
9. // creation of the 3 variables
10. int var1, var2, var3;
11.
12. // initialization
13. println("Enter a value:");
14. var1 = input();
15. println("Enter a value:");
16. var2 = input();
17. println("Enter a value:");
18. var3 = input();
19.
20. // sum operation
21. int var4 = var1 + var2;
22.
23. // multiplication operation
24. int var5 = var2 * var3;
25.
26. // variables printing
27. println("Value1: "+var1);
28. println("Value2: "+var2);
29. println("Value3: "+var3);
30. println("Value4: "+var4);
31. println("Value5: "+var5);
32.}
Now we are going to separate (modularize) the initialization operations, which prompts the user for values (lines 13 to 18 of the code above).
Note that the initialization of each variable consists of printing the message “Enter a value:” and requesting the value typed on the keyboard by the command “input()”. For this modularization, we can create a function called “enterValue”. The code below shows this function.
1.int enterValue(){
2. println("Enter a value:");
3. int var = input();
4. return var;
5.}
The declaration of this function seems more complicated than that of the previous section. Here we include the word int in place of void (line 1 of the code above) and the word return (line 4 of the code above).
The term int refers to the return type of the function. It means that this function will return (for whoever invoked it) a integer type value. And this return is performed at the end of the function by the word return.
Thus, the main code of the algorithm is as follows:
1.void welcome(){
2. println("Hello");
3. println("Welcome to the System!");
4.}
5.
6.int enterValue(){
7. println("Enter a value:");
8. int var = input();
9. return var;
10.}
11.
12.void main(){
13. welcome();
14.
15. // creation of the 3 variables
16. int var1, var2, var3;
17.
18. var1 = enterValue();
19. var2 = enterValue();
20. var3 = enterValue();
21.
22. // sum operation
23. int var4 = var1 + var2;
24.
25. // multiplication operation
26. int var5 = var2 * var3;
27.
28. // variables printing
29. println("Value1: "+var1);
30. println("Value2: "+var2);
31. println("Value3: "+var3);
32. println("Value4: "+var4);
33. println("Value5: "+var5);
34.}
It is possible to observe in lines 6 to 10 of the code above the declaration of the function “enterValue()”.
You can also see on lines 18 to 20 of the code above that the request commands have been removed. In its place, the invocation commands for the function “enterValue()” were placed. The difference here is that these invocations are being attributed to the variables var1, var2 and var3. This is possible because the function “enterValue()” has a return, which is sent back and assigned to variables var1, var2 and var3.
See the animation below for a simulation of the execution, line by line, of the algorithm with the function “enterValue()”.
Creating a function with Parameters
We have already learned how to create simple functions (which return nothing) and functions with return (which return values). Now let’s learn how to create functions with parameters (which send values).
To do this, let’s go back to the same algorithm as before, from where we left off:
1.void welcome(){
2. println("Hello");
3. println("Welcome to the System!");
4.}
5.
6.int enterValue(){
7. println("Enter a value:");
8. int var = input();
9. return var;
10.}
11.
12.void main(){
13. welcome();
14.
15. // creation of the 3 variables
16. int var1, var2, var3;
17.
18. var1 = enterValue();
19. var2 = enterValue();
20. var3 = enterValue();
21.
22. // sum operation
23. int var4 = var1 + var2;
24.
25. // multiplication operation
26. int var5 = var2 * var3;
27.
28. // variables printing
29. println("Value1: "+var1);
30. println("Value2: "+var2);
31. println("Value3: "+var3);
32. println("Value4: "+var4);
33. println("Value5: "+var5);
34.}
We are going to modularize the sum operation. For this, we can create a function called “sum”. This function will only have the purpose of adding two values sent by parameter (between the parentheses ‘(‘ ‘)’ of the function).
See the code for this function below:
1.int sum(int a, int b){
2. int var = a + b;
3. return var;
4.}
The code above illustrates the creation of a function that has the ability to receive parameters. Note in line 1 of the code above that there is, within parentheses, the declaration of the two integer variables “a” and “b”. These variables are the parameters of the function. They will receive the values to be added when the “sum()” function is invoked. Below is an example of how we can invoke this function and pass the parameters:
1.int sum(int a, int b){
2. int var = a + b;
3. return var;
4.}
5.
6.void main(){
7. int result = sum(5, 8);
8.}
Line 7 of the code above shows the invocation of the “sum()” function with the passing of the parameters. Values 5 and 8 are passed, respectively, to the variables “a” e “b” (line 1 of the code above). After that, the values are added (line 2 of the code above), resulting in the value 13. Then, the value 13 is returned (line 3 of the code above). Finally, this returned value 13 is assigned to the variable “result” (line 7 of the code above).
Click on the animation below to see the simulation of the code execution above:
NOTE: when a function has parameters in its declaration, passing these parameters is mandatory during its invocation!
Following the same reasoning, we can create a function to perform the multiplication:
1.int multiplies(int a, int b){
2. int var = a * b;
3. return var;
4.}
See below the complete algorithm with all operations already modularized and in several different programming languages:
1.import java.util.Scanner;
2.class Main {
3. public static void welcome(){
4. System.out.println("Hello");
5. System.out.println("Welcome to the System!");
6. }
7. public static int enterValue(){
8. System.out.println("Enter a value:");
9. Scanner sc = new Scanner(System.in);
10. int var = sc.nextInt();
11. return var;
12. }
13. public static int sum(int a, int b){
14. int var = a + b;
15. return var;
16. }
17. public static int multiplies(int a, int b){
18. int var = a * b;
19. return var;
20. }
21. public static void main(String[] args) {
22. welcome();
23. // creation of the 3 variables
24. int var1, var2, var3;
25. // request for values
26. var1 = enterValue();
27. var2 = enterValue();
28. var3 = enterValue();
29. // math operations
30. int var4 = sum(var1, var2);
31. int var5 = multiplies(var2, var3);
32. // variables printing
33. System.out.println("Value1: "+var1);
34. System.out.println("Value2: "+var2);
35. System.out.println("Value3: "+var3);
36. System.out.println("Value4: "+var4);
37. System.out.println("Value5: "+var5);
38. }
39.}
1.#include <stdio.h>
2.void welcome(){
3. printf("Hello\n");
4. printf("Welcome to the System!\n");
5.}
6.int enterValue(){
7. printf("Enter a value:\n");
8. int var;
9. scanf("%d",&var);
10. return var;
11.}
12.int sum(int a, int b){
13. int var = a + b;
14. return var;
15.}
16.int multiplies(int a, int b){
17. int var = a * b;
18. return var;
19.}
20.int main(void) {
21. welcome();
22. // creation of the 3 variables
23. int var1, var2, var3;
24. // request for values
25. var1 = enterValue();
26. var2 = enterValue();
27. var3 = enterValue();
28. // math operations
29. int var4 = sum(var1, var2);
30. int var5 = multiplies(var2, var3);
31. // variables printing
32. printf("Value1: %d\n",var1);
33. printf("Value2: %d\n",var2);
34. printf("Value3: %d\n",var3);
35. printf("Value4: %d\n",var4);
36. printf("Value5: %d\n",var5);
37.}
1.#include <iostream>
2.void welcome(){
3. std::cout << "Hello\n";
4. std::cout << "Welcome to the System!\n";
5.}
6.int enterValue(){
7. std::cout << "Enter a value:\n";
8. int var;
9. std::cin >> var;
10. return var;
11.}
12.int sum(int a, int b){
13. int var = a + b;
14. return var;
15.}
16.int multiplies(int a, int b){
17. int var = a * b;
18. return var;
19.}
20.int main() {
21. welcome();
22. // creation of the 3 variables
23. int var1, var2, var3;
24. // request for values
25. var1 = enterValue();
26. var2 = enterValue();
27. var3 = enterValue();
28. // math operations
29. int var4 = sum(var1, var2);
30. int var5 = multiplies(var2, var3);
31. // variables printing
32. std::cout << "Value1: " << var1 << std::endl;
33. std::cout << "Value2: " << var2 << std::endl;
34. std::cout << "Value3: " << var3 << std::endl;
35. std::cout << "Value4: " << var4 << std::endl;
36. std::cout << "Value5: " << var5 << std::endl;
37.}
1.using System;
2.class MainClass {
3. public static void welcome(){
4. Console.WriteLine("Hello");
5. Console.WriteLine("Welcome to the System!");
6. }
7. public static int enterValue(){
8. Console.WriteLine("Enter a value:");
9. int var = Convert.ToInt32(Console.ReadLine());
10. return var;
11. }
12. public static int sum(int a, int b){
13. int var = a + b;
14. return var;
15. }
16. public static int multiplies(int a, int b){
17. int var = a * b;
18. return var;
19. }
20. public static void Main (string[] args) {
21. welcome();
22. // creation of the 3 variables
23. int var1, var2, var3;
24. // request for values
25. var1 = enterValue();
26. var2 = enterValue();
27. var3 = enterValue();
28. // math operations
29. int var4 = sum(var1, var2);
30. int var5 = multiplies(var2, var3);
31. // variables printing
32. Console.WriteLine("Value1: "+var1);
33. Console.WriteLine("Value2: "+var2);
34. Console.WriteLine("Value3: "+var3);
35. Console.WriteLine("Value4: "+var4);
36. Console.WriteLine("Value5: "+var5);
37. }
38.}
1.def welcome():
2. print("Hello")
3. print("Welcome to the System!")
4.def enterValue():
5. var = int(input("Enter a value:"))
6. return var
7.def sum(a, b):
8. var = a + b
9. return var
10.def multiplies(a, b):
11. var = a * b
12. return var
13.welcome();
14.# request for values
15.var1 = enterValue()
16.var2 = enterValue()
17.var3 = enterValue()
18.# math operations
19.var4 = sum(var1, var2)
20.var5 = multiplies(var2, var3)
21.# variables printing
22.print("Value1: "+str(var1))
23.print("Value2: "+str(var2))
24.print("Value3: "+str(var3))
25.print("Value4: "+str(var4))
26.print("Value5: "+str(var5))
If you are already familiar with all the subjects of basic programming, you are certainly prepared to study more advanced programming topics, such as Object Oriented Programming or Data Structures.
The important thing is that you continue to learn. Programming can provide you with wonderful possibilities.
David Santiago
Master in Systems and Computing. Graduated in Information Systems. Professor of Programming Language, Algorithms, Data Structures and Development of Digital Games.